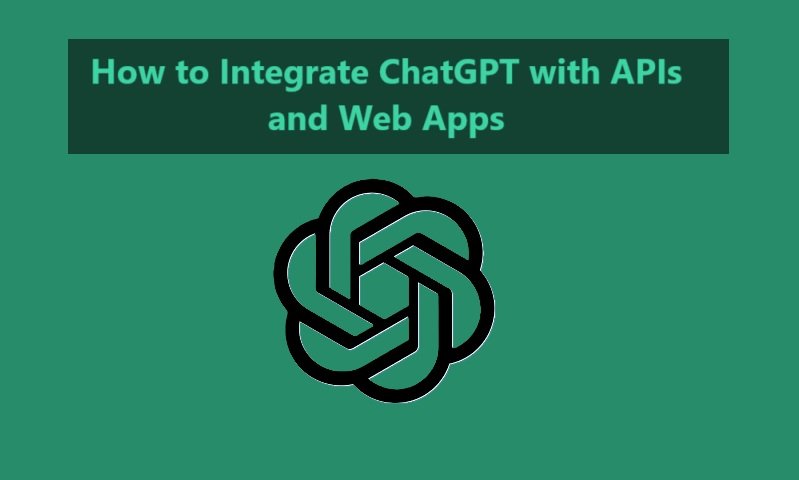
How to Integrate ChatGPT with APIs and Web Apps
ChatGPT is a powerful AI model that can be integrated into web applications and APIs to automate tasks, generate content, assist users, and more. If you are a developer or business owner looking to enhance your web app with AI, this guide will walk you through the steps to integrate ChatGPT with APIs and web applications in a simple and detailed manner.
1. Understanding API Integration
APIs (Application Programming Interfaces) allow different software applications to communicate with each other. OpenAI provides an API for ChatGPT, which lets developers integrate it into their applications to generate text, answer queries, and perform various AI-powered tasks.
2. Getting Access to OpenAI’s ChatGPT API
Before you start integration, you need access to the OpenAI API:
- Go to OpenAI’s official website (https://openai.com).
- Sign up or log in to your account.
- Navigate to the API section and apply for API access.
- Once approved, get your API key from the dashboard.
This API key is essential for making requests to the ChatGPT model.
3. Setting Up Your Development Environment
To integrate ChatGPT into your web app, you need to set up a development environment. Follow these steps:
- Install Python (if not already installed) or use a language like JavaScript (Node.js).
- Install necessary libraries: For Python:
pip install openai requests
For JavaScript (Node.js):npm install openai axios
4. Making API Calls to ChatGPT
Once your environment is set up, you can send a request to the ChatGPT API.
Example using Python:
import openai
openai.api_key = "your-api-key-here"
response = openai.ChatCompletion.create(
model="gpt-4",
messages=[
{"role": "system", "content": "You are a helpful assistant."},
{"role": "user", "content": "How do I integrate ChatGPT?"}
]
)
print(response["choices"][0]["message"]["content"])
Example using JavaScript (Node.js):
const { Configuration, OpenAIApi } = require("openai");
const configuration = new Configuration({
apiKey: "your-api-key-here",
});
const openai = new OpenAIApi(configuration);
async function chatWithGPT() {
const response = await openai.createChatCompletion({
model: "gpt-4",
messages: [{ role: "user", content: "How do I integrate ChatGPT?" }],
});
console.log(response.data.choices[0].message.content);
}
chatWithGPT();
5. Integrating ChatGPT into a Web Application
If you want to integrate ChatGPT into a web app, you can create a simple frontend + backend setup.
Frontend (HTML + JavaScript Example)
<!DOCTYPE html>
<html>
<head>
<title>ChatGPT Web App</title>
</head>
<body>
<h2>Ask ChatGPT</h2>
<textarea id="userInput" placeholder="Type your question here..."></textarea>
<button onclick="askChatGPT()">Send</button>
<p id="response"></p>
<script>
async function askChatGPT() {
const userMessage = document.getElementById("userInput").value;
const response = await fetch("/ask", {
method: "POST",
headers: { "Content-Type": "application/json" },
body: JSON.stringify({ message: userMessage })
});
const data = await response.json();
document.getElementById("response").innerText = data.reply;
}
</script>
</body>
</html>
Backend (Node.js + Express Example)
const express = require("express");
const cors = require("cors");
const { Configuration, OpenAIApi } = require("openai");
const app = express();
app.use(express.json());
app.use(cors());
const configuration = new Configuration({ apiKey: "your-api-key-here" });
const openai = new OpenAIApi(configuration);
app.post("/ask", async (req, res) => {
const userMessage = req.body.message;
const response = await openai.createChatCompletion({
model: "gpt-4",
messages: [{ role: "user", content: userMessage }],
});
res.json({ reply: response.data.choices[0].message.content });
});
app.listen(3000, () => console.log("Server running on port 3000"));
Now, running this backend with the frontend will allow users to interact with ChatGPT from a web interface.
6. Best Practices for API Integration
- Secure Your API Key: Never expose your API key in client-side code.
- Optimize API Calls: Implement caching and rate limits to avoid excessive requests.
- Handle Errors Gracefully: Use try-catch blocks to manage API failures.
- Monitor Usage: Keep track of API usage to avoid hitting limits.
- Ensure User Privacy: Avoid sending sensitive data to AI models.
7. Conclusion
Integrating ChatGPT with APIs and web applications is a powerful way to enhance user interaction, automate tasks, and build AI-driven applications. By following the steps outlined in this guide, you can set up ChatGPT for your web app efficiently. Whether you’re building a chatbot, an assistant, or a content generation tool, the flexibility of OpenAI’s API makes it a valuable addition to modern web applications.